Creating JavaScript chess board is fairly simple. All you need to do is create 64 squares using div elements with alternating background colors, and you’re pretty much done. Here is the expected end result:

In this article you will find:
P.S. another good chess move is to learn how to double your salary as a programmer
Empty Chess Board
Here is a full JavaScript code (also explained line-by-line below)
<script>
window.onload = function() {
const board = document.createElement('div');
board.classList.add("board");
document.body.appendChild(board);
for (let row = 1; row <= 8; row++) {
for (let column = 1; column <= 8; column++) {
const square = document.createElement('div');
square.classList.add("square");
if (
((row%2 === 0 && column%2 === 1)) ||
((row%2 === 1 && column%2 === 0))
) {
square.classList.add("dark");
}
board.appendChild(square);
}
}
}
</script>
This is a complete HTML code because the browser generates default elements like <body> automatically.
window.onload function is called once the browser loaded all the elements (like <body> and only then we start to make changes in the HTML).
In the next 3 lines, we’re programmatically creating a container for all the squares called the board. We assign a board class (which will help us later with styling in CSS). And the last line adds the board element to the HTML (inside the <body> element).
for loops are going through all 8 rows and 8 columns. Inside the loops we’re assigning the CSS classes to the squares for easier styling later. And lastly, we’re adding them to the chess board.
The only complex part is this part here:
if (
((row%2 === 0 && column%2 === 1)) ||
((row%2 === 1 && column%2 === 0))
) {
square.classList.add("dark");
}
If chess board squares just alternated between dark and light squares, out job would be easy. Something like this:
if (row%2 === 0) square.classList.add("dark");
But, this is not the case. When we create the 64 squares, we put them in one long array. However, you will notice that the last tile in the top row is a dark square. And the very next square in our array (first tile in the second row) is also a dark square.
Basically, what this means is – we need to change the dark-light color alteration sequence for every new row. Top row starts with a light square and second row starts with a dark square and so on…
And this if statement:
- assigns dark square on every odd column if we are in an even row
- assigns dark square on every even column if we are in an odd row
And with this our JavaScript is done.
CSS Styling
Now we need to add a little bit of CSS to make our chess board visible:
<style>
* {
margin: 0;
padding 0;
}
.board {
width: 90vmin;
height: 90vmin;
border: 10px solid #333333;
margin: 0 auto;
margin-top: 4vmin;
}
.square {
min-width: 12.5%;
min-height: 12.5%;
outline: 1px solid gray;
float: left;
background-color: lightgray;
}
.dark {
background-color: #bb0000;
}
</style>
* – first we remove all the default HTML styling from all the elements.
.board – then we define width and height of out board. It is set to 90% of the size of the smaller screen dimension.
For example, vw (viewport width) is the width of your screen and vh (viewport height) is the height of your screen. vmin is the smaller value of the two. This value is important because we want to fit our square board into a screen and we’re limited by the smaller side of the screen.
90vmin is 90% of the smaller side of the screen because I wanted to leave some space between the board and the edge of the screen (it looks nicer).
margin: 0 auto; – positions the chess board nicely at the center of the screen (horizontal centering). For vertical centering I just used a little bit of margin and hoped for the best 🙂
.square has the dimension set to 12.5% because 100% divided by 8 (because there are 8 squares in a row) equals 12.5
outline is used rather than border because border adds width to the element. This means our 8 x 12.5% + the widths of the border would be over 100% and 8 squares wouldn’t fit into our board. In short, our board would fall apart.
float: left; is used because by default <div> elements would just go into the new line. So we need this styling to make those elements arrange into a row.
Board With Chess Pieces
Now that we created the board, adding pieces is fairly simple. All we need to do is to add some code above this line:
board.appendChild(square);
The key thing to know is that HTML (and your browser) has standardized codes for displaying chess pieces like this: ♔ ♕ ♖ ♗ ♘ ♙
First thing we will do is to add the pawns to the 2nd and 7th row (rank).
if (row === 2) square.innerHTML = "♟";
if (row === 7) square.innerHTML = "♙";
2nd row contains the code for black pawns and 7th row contains the code for white pawns.
After that we need to add the chess pieces:
const blackPieces = [
'♜', '♞', '♝', '♛',
'♚', '♝', '♞', '♜'];
const whitePieces = [
'♖', '♘', '♗', '♕',
'♔', '♗', '♘', '♖'];
if (row === 1) {
square.innerHTML = blackPieces[column-1];
}
if (row === 8) {
square.innerHTML = whitePieces[column-1];
}
Black pieces are added to the top row and white pieces are added to the bottom row.
Currently they look very small in comparison to the board, positioned in the corner, but a little CSS can certainly fix that. Just add the following 2 lines to .square and you’re done:
line-height: 100%;
font-size: 10vmin;
And I also changed .dark class CSS because it looks better with the pieces on the board:
.dark {
background-color: gold;
}
Here is the final result:
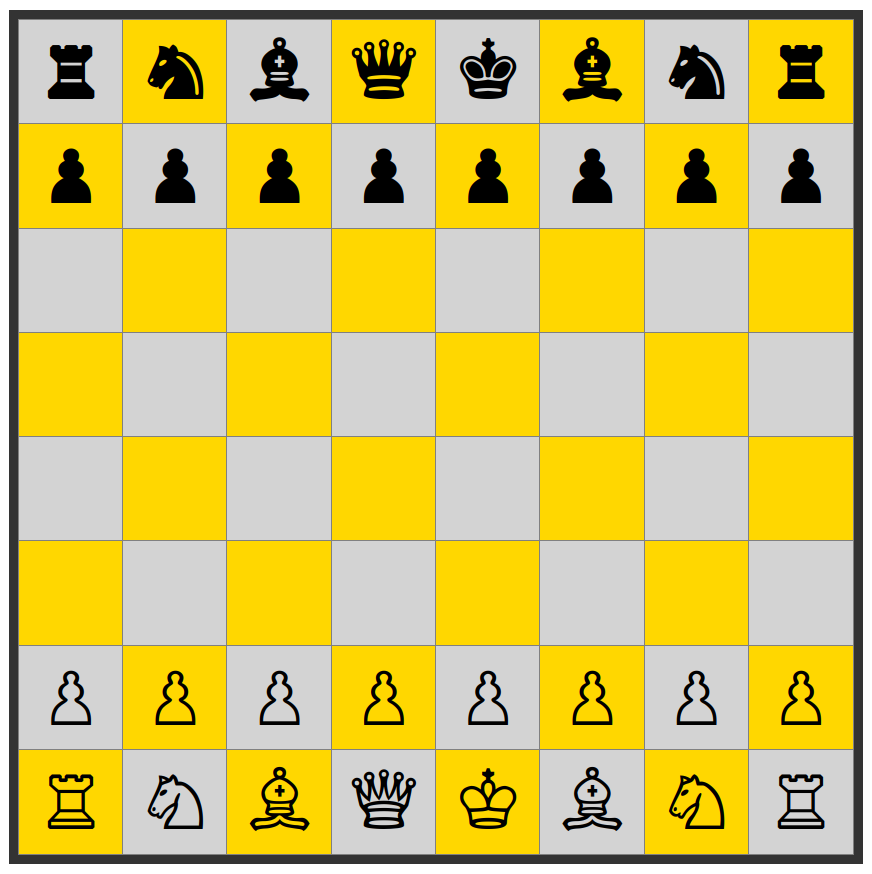
P.S. in chess and in life a player who makes the best moves – wins. If you knew how to double your salary as a programmer (without working any harder), you would win more frequently.